ionic是一个基于Angular、Cordova的一个开源框架,它提供了相关的UI库,ionic2中对ionic1进行了更多的优化,让ionic的运行速度,执行效率提高了不少。选择ionic开发web app是个不错的想法。下面就来说下ionic2中的loading效果的实现吧!本文使用TypeScript进行对ionic2的开发,如果不了解微软的TypeScript的,请自己google一下哦!
引入LoadingController
我们要使用LoadingController,就必须先引入它,直接使用下面的代码即可实现
import { LoadingController } from ‘ionic-angular’;
引入后,我们就需要在constructor中声明了,如下:
constructor(public loadingCtrl: LoadingController){
//……..在这里执行loading效果或者设置loading的图标、css等
}
看上去是很简单哦,要使用这个loading,那么我们得先创建它。
创建create()
直接可以使用create方法,create方法中又包括了以下几个属性:
spinner(设置loading的SVG图片)
content(设置loading的提示文字或内容)
loadingSpinner(这个我暂时没有理解到底是做什么的)
移除(也可以叫解雇)dismiss()
移除是当我们的loading完成后,自动移除当前loading,移除后,就无法再次在当前组件中使用它了。
加载指示器可以在特定时间后通过传递毫秒数来自动关闭,以duration在加载选项中显示它。默认情况下,加载指示器即使在页面更改期间也会显示,但可以通过设置dismissOnPageChange来禁用 true。要在创建后关闭加载指示器,请调用dismiss()“加载”实例上的方法。onDidDismiss可以调用该 功能,在加载指示灯关闭后执行动作。
使用LoadingController
默认UI的loading
1 | constructor(public loadingCtrl: LoadingController) { |
2 | |
3 | } |
4 | |
5 | presentLoadingDefault() { |
6 | let loading = this.loadingCtrl.create({ |
7 | content: 'Please wait...'//设置loading时显示的文字 |
8 | }); |
9 | |
10 | loading.present(); |
11 | |
12 | setTimeout(() => {//设置loading的时间 |
13 | loading.dismiss(); |
14 | }, 5000); |
15 | } |
自定义loading
1 | presentLoadingCustom() { |
2 | let loading = this.loadingCtrl.create({ |
3 | spinner: 'hide', |
4 | content: ` |
5 | <div class="custom-spinner-container"> |
6 | <div class="custom-spinner-box"><img src=""></div> //自定义loading图片(可以是svg或者gif)和div |
7 | </div>`, |
8 | duration: 5000 |
9 | }); |
10 | |
11 | loading.onDidDismiss(() => { |
12 | console.log('Dismissed loading'); |
13 | }); |
14 | |
15 | loading.present(); |
16 | } |
这样就定义好一个属于自己的loading了,但是问题来了,你在loading的时候,总是有一层白色背景在你的loading图片后面,如下图:
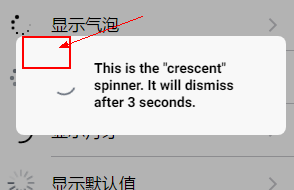
如果是在IOS上,这样的效果很原生的,非常漂亮,但是如果你不想要这个白色的背景,那是可以通过修改scss变量来进行设置的。
首先我们打开theme文件夹下的variables.scss,给他设置如下变量,即可去除背景以及背景阴影了
1 | $loading-md-background: none; |
2 | $loading-md-border-radius: 0; |
3 | $loading-md-box-shadow-color:rgba(158, 158, 158,0); |
4 | $loading-md-box-shadow:rgba(158, 158, 158,0); |
5 | $loading-ios-background: none; |
6 | $loading-ios-border-radius: 0; |
7 | $loading-wp-background: none; |
8 | $loading-wp-border-radius: 0; |
一些你肯定用得到的参数及scss变量
create(opts)
Create a loading indicator. See below for options.
Param Type Details
opts LoadingOptions
Loading options
Returns: Loading
Returns a Loading Instance
Advanced
Loading options
Option Type Description
spinner string The name of the SVG spinner for the loading indicator.
content string The html content for the loading indicator.
cssClass string Additional classes for custom styles, separated by spaces.
showBackdrop boolean Whether to show the backdrop. Default true.
dismissOnPageChange boolean Whether to dismiss the indicator when navigating to a new page. Default false.
duration number How many milliseconds to wait before hiding the indicator. By default, it will show until dismiss() is called.
Sass Variables
iOS Material Design Windows Platform
Property Default Description
$loading-ios-padding 24px 34px
Padding of the loading wrapper
$loading-ios-max-width 270px
Max width of the loading wrapper
$loading-ios-max-height 90%
Maximum height of the loading wrapper
$loading-ios-border-radius 8px
Border radius of the loading wrapper
$loading-ios-text-color #000
Text color of the loading wrapper
$loading-ios-background #f8f8f8
Background of the loading wrapper
$loading-ios-content-font-weight bold
Font weight of the loading content
$loading-ios-spinner-color #69717d
Color of the loading spinner
$loading-ios-spinner-ios-color $loading-ios-spinner-color
Color of the ios loading spinner
$loading-ios-spinner-bubbles-color $loading-ios-spinner-color
Color of the bubbles loading spinner
$loading-ios-spinner-circles-color $loading-ios-spinner-color
Color of the circles loading spinner
$loading-ios-spinner-crescent-color $loading-ios-spinner-color
Color of the crescent loading spinner
$loading-ios-spinner-dots-color $loading-ios-spinner-color
Color of the dots loading spinner
更多可以查看官方文档:http://ionicframework.com/docs/api/components/loading/LoadingController/